c++ 教程:数据类型
大约 13 分钟
变量定义(Variable Declarations)
type variable = value;
type variable {value}; C++11
type variable {value}; C++11
// declare & initialize 'i'"
int i = 1;
// print i's value:
cout << i << '\n';
int j {5};
cout << j << '\n';
int i = 1;
// print i's value:
cout << i << '\n';
int j {5};
cout << j << '\n';
基本数据类型(Fundamental Types)
布尔类型(Booleans)
bool b1 = true; bool b2 = false;
字符类型(Characters)
char c = 'A'; // character literal char a = 65; // same as above
有符号整数(Signed Integers)
n bits ⇒ values ∈ [-2(n-1), 2(n-1)-1]
short s = 7; int i = 12347; long l1 = -7856974990L; long long l2 = 89565656974990LL; // ' digit separator C++14 long l3 = 512'232'697'499;
无符号整数(Unsigned Integers)
n bits ⇒ values ∈ [0, 2n-1]
unsigned u1 = 12347U; unsigned long u2 = 123478912345UL; unsigned long long u3 = 123478912345ULL; // non-decimal literals unsigned x = 0x4A; // hexadecimal unsigned b = 0b10110101; // binary C++14
浮点类型
float f = 1.88f; double d1 = 3.5e38; long double d2 = 3.5e38L; C++11 // ' digit separator C++14 double d3 = 512'232'697'499.052;
数字表示法(Number Representations)
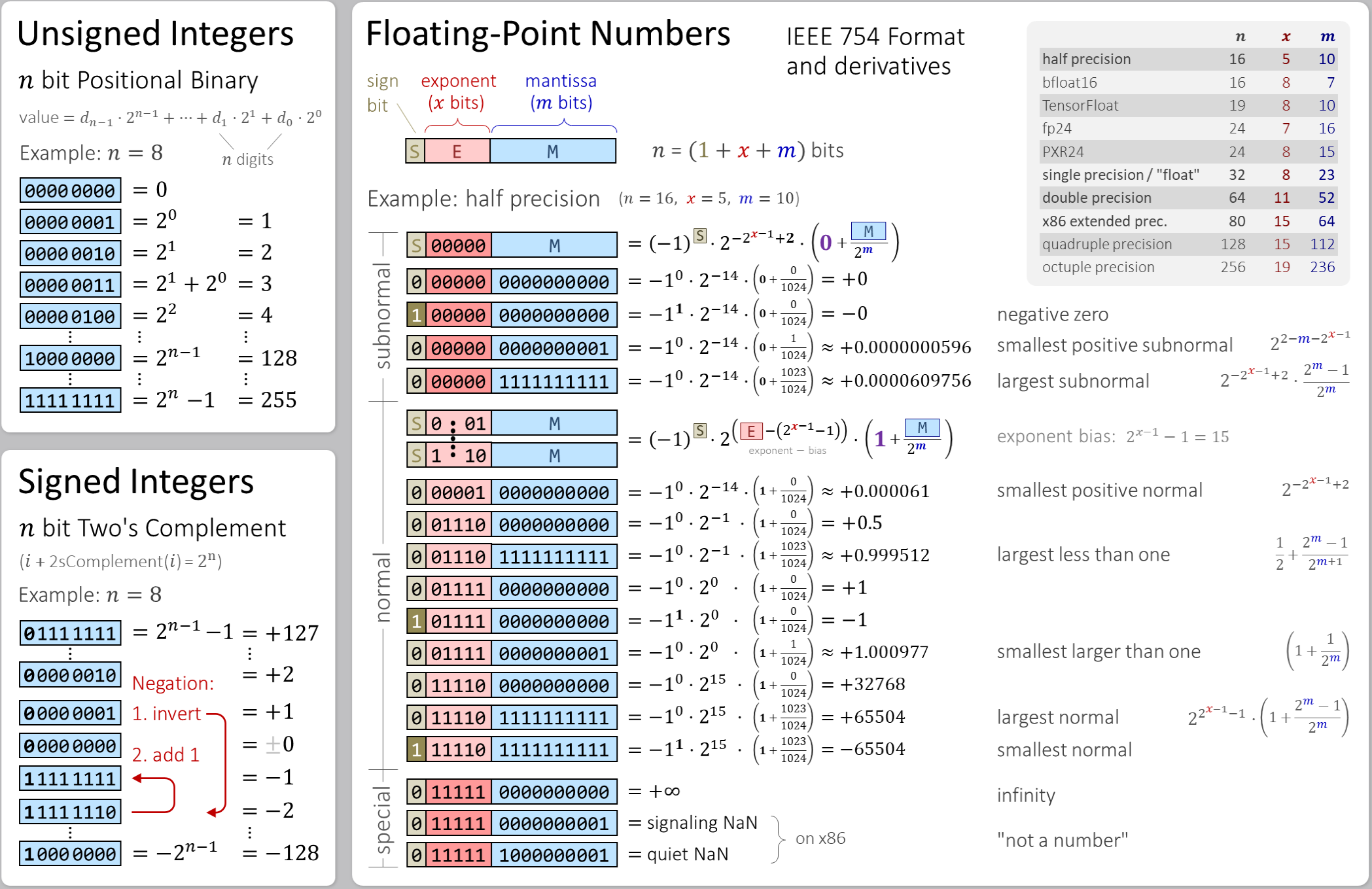
类型的内存大小(Memory Sizes of Types)
cout << sizeof(char); // 1 cout << sizeof(bool); // 1 cout << sizeof(short); // 2 cout << sizeof(int); // 4 cout << sizeof(long); // 8
// number of bits in a char cout << CHAR_BIT; // 8
char c = 'A'; bool b = true; int i = 1234; long l = 12; short s = 8;
数值限制(std::numeric_limits)
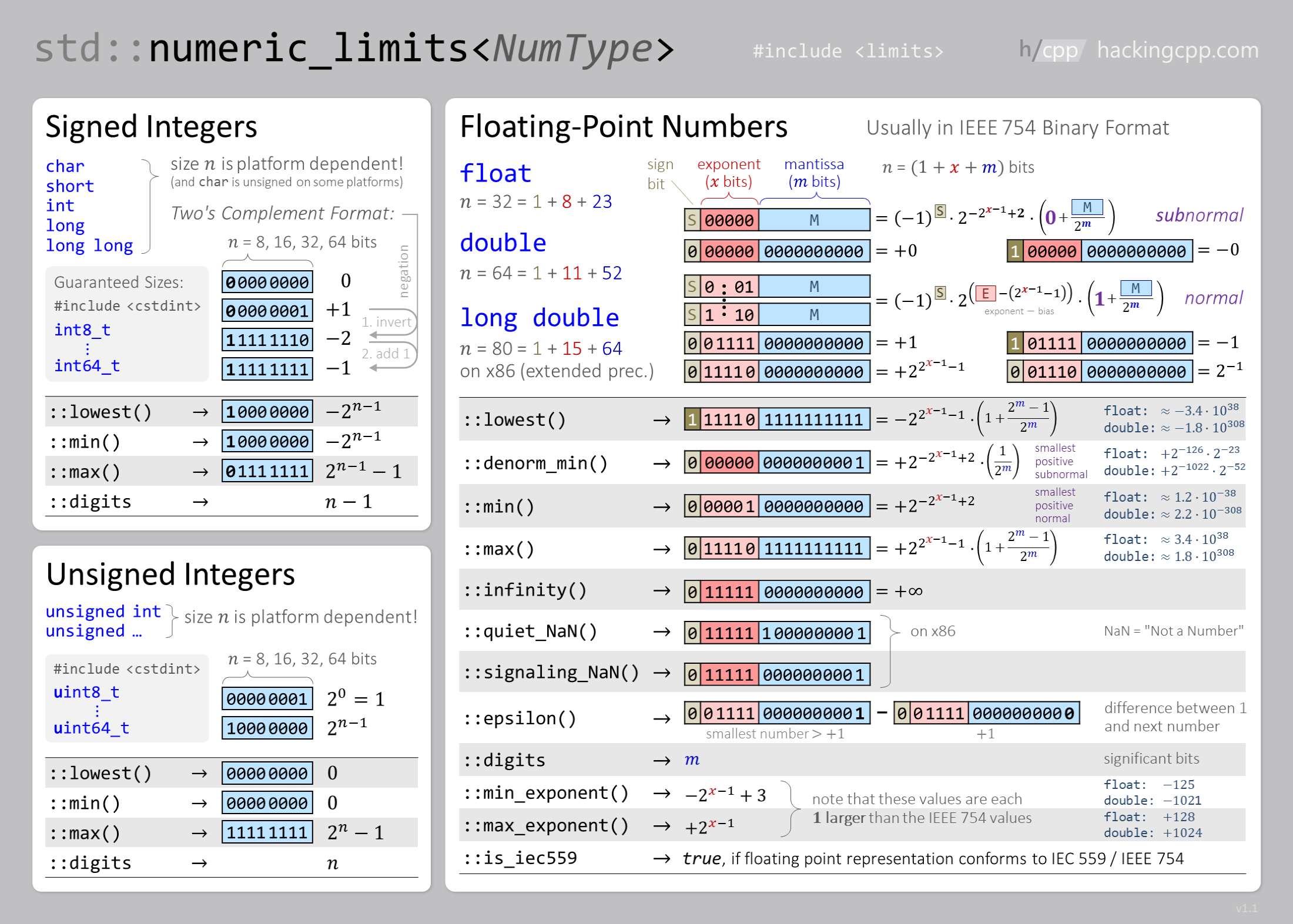
算数运算(Arithmetic Operations )
Operators
int a = 4; 设置变量 a 值为 4 int b = 3; 设置变量 b 值为 3
a = a + b; a: 7 add a += b; a: 10 a = a - b; a: 7 subtract a -= b; a: 4 a = a * b; a: 12 multiply a *= b; a: 36 a = a / b; a: 12 divide a /= b; a: 4 a = a % b; a: 1 remainder of division (modulo)
自增/自减(Increment/Decrement )
int a = 4; a: 4 int b = 3; b: 3
b = a++; a: 5 b: 4 b = ++a; a: 6 b: 6 b = --a; a: 5 b: 5 b = a--; a: 4 b: 5
比较运算(Comparisons)
2路比较(2-way Comparisons)
比较结果为 true 或者 false
int x = 10; int y = 5; result operator
bool b1 = x == 5; false equals bool b2 = (x != 6); true not equal bool b3 = x > y; true greater bool b4 = x < y; false smaller bool b5 = y >= 5; true greater/equal bool b6 = x <= 30; true smaller/equal
3路比较(3-Way Comparisons)
比较 2 个对象的相对顺序
(a <==> b) < 0 if a < b (a <==> b) > 0 if a > b (a <==> b) == 0 if a and b are equal/equivalent
布尔逻辑(Boolean Logic)
操作符(Operators)
bool a = true; bool b = false;
bool c = a && b; // false logical AND bool d = a || b; // true logical OR bool e = !a; // false logical NOT
Alternative Spellings: bool x = a and b; // false bool y = a or b; // true bool z = not a; // false
转化成bool(Conversion to bool)
bool f = 12; // true (int → bool) bool g = 0; // false (int → bool) bool h = 1.2; // true (double → bool)
位运算(Bitwise Operations)
位逻辑运算(Bitwise Logic)
memory bits: std::uint8_t a = 6; 0000 0110 std::uint8_t b = 0b00001011; 0000 1011
std::uint8_t c1 = (a & b); // 2 0000 0010 std::uint8_t c2 = (a | b); // 15 0000 1111 std::uint8_t c3 = (a ^ b); // 13 0000 1101 std::uint8_t c4 = ~a; // 249 1111 1001 std::uint8_t c5 = ~b; // 244 1111 0100
// test if int is even/odd: result: bool a_odd = a & 1; 0 ⇒ false bool a_even = !(a & 1); 1 ⇒ true
位移运算(Bitwise Shifts)
memory bits: std::uint8_t a = 1; 0000 0001
a <<= 6; // 64 0100 0000 a >>= 4; // 4 0000 0100
std::uint8_t b1 = (1 << 1); // 2 0000 0010 std::uint8_t b2 = (1 << 2); // 4 0000 0100 std::uint8_t b3 = (1 << 4); // 16 0001 0000
枚举类型(Enumerations)
定义(Defining)
enum class day { mon, tue, wed, thu, fri, sat, sun }; day d = day::mon; d = day::tue; // ✔ d = wed; // ✖ COMPILER ERROR: 'wed' only known in day's scope
底层数据类型(Underlying Type Of Enumerations)
// 7 values ⇒ char should be enough enum class day : char { mon, tue, wed, thu, fri, sat, sun }; // less than 10,000 ⇒ short should be enough enum class language_ISO639 : short { abk, aar, afr, aka, amh, ara, arg, … };
类型系统(Type System)
定义常量(Declare Constants)
Type const variable_name = value; 变量值一旦赋值就不可以修改 变量值可以运行时初始化
int i = 0; cin >> i; int const k = i; // "int constant" k = 5; // ✖ COMPILER ERROR: k is const!
类型别名(Type Aliases)
using real = double; using ullim = unsigned long; using index_vector = std::uint_least64_t;
自动推导(Type Deduction: auto)
auto i = 2; int auto u = 56u; unsigned int auto d = 2.023; double auto f = 4.01f; float auto l = -78787879797878l; long int
auto x = 0 * i; x: int auto y = i + d; y: double auto z = f * d; z: double